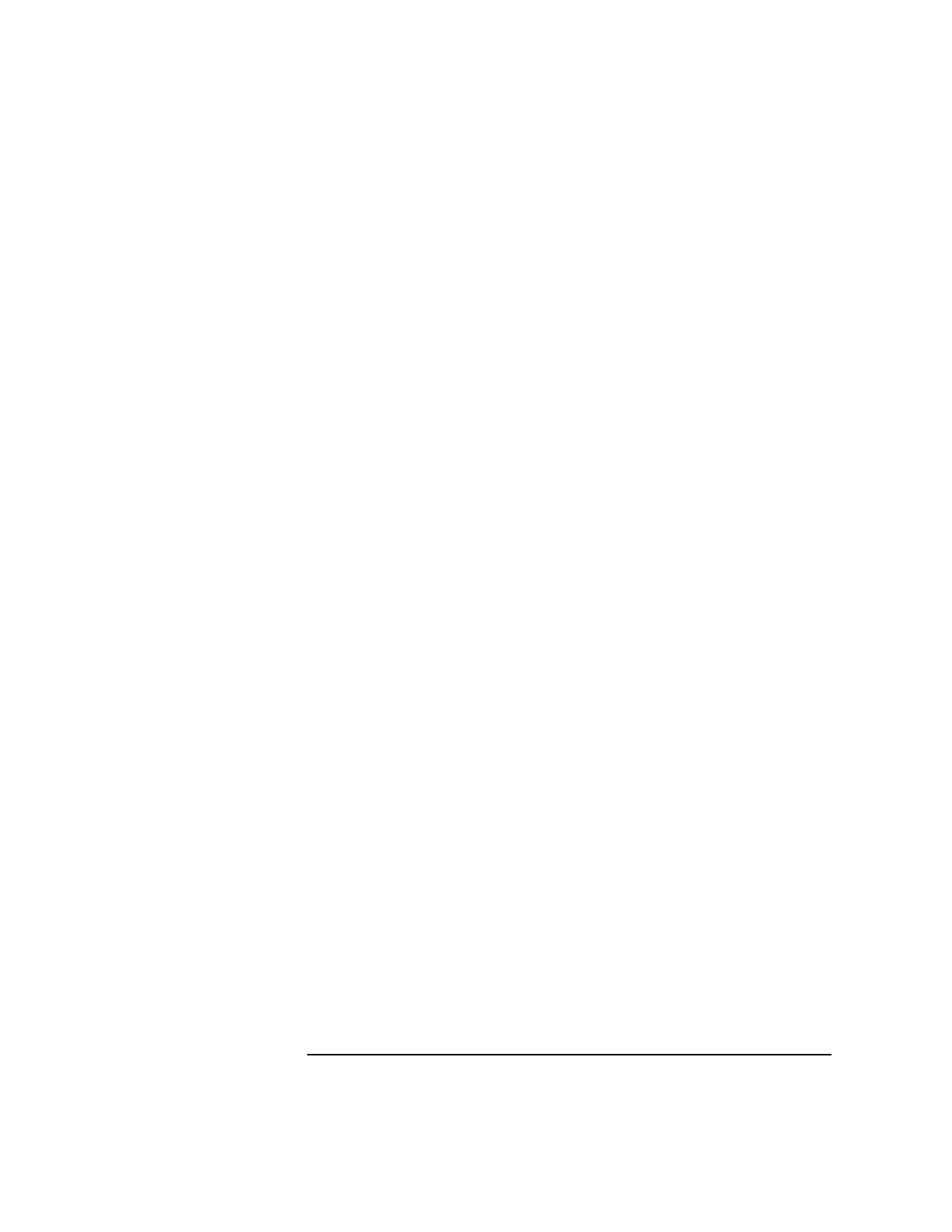
Chapter 2 29
LLA and DLPI Example Programs
DLPI Example Program
/*********************************************************************
main
*********************************************************************/
main() {
int send_fd, recv_fd; /* file descriptors */
u_char sdlsap[20]; /* sending DLSAP */
u_char rdlsap[20]; /* receiving DLSAP */
int sdlsap_len, rdlsap_len; /* DLSAP lengths */
int i, j, recv_len;
/*
PART 1 of program. Demonstrate connectionless data
transfer with LLC SAP header.
*/
/*
First, we must open the DLPI device file, /dev/dlpi, and attach
to a PPA. attach() will open /dev/dlpi, find the first PPA
with the DL_HP_PPA_INFO primitive, and attach to that PPA.
attach() returns the file descriptor for the stream. Here we
do an attach for each file descriptor.
*/
send_fd = attach();
recv_fd = attach();
/*
Now we have to bind to a IEEESAP. We will ask for connectionless
data link service with the DL_CLDLS service mode. Since we are
connectionless, we will not have any incoming connections so we
set max_conind to 0. bind() will return our local DLSAP and its
length in the last two arguments we pass to it.
*/
bind(send_fd, SEND_SAP, 0, DL_CLDLS, sdlsap, &sdlsap_len);
bind(recv_fd, RECV_SAP, 0, DL_CLDLS, rdlsap, &rdlsap_len);
/* print the DLSAPs we got back from the binds */
print_dlsap(”sending DLSAP = ”, sdlsap, sdlsap_len);
print_dlsap(”receiving DLSAP = ”, rdlsap, rdlsap_len);
/*
Time to send some data. We'll send 5 data packets in sequence.
*/
for(i = 0; i < 5; i++) {
/* send (i+1)*10 data bytes with the first byte = i */
data_area[0] = i;
/* Initialize data area */
for (j = 1; j < (i+1)*10; j++)
data_area[j] = ”a”;
print_dlsap(”sending data to ”,rdlsap, rdlsap_len);
send_data(send_fd, rdlsap, rdlsap_len, (i + 1) * 10);
/* receive the data packet */
recv_len = recv_data(recv_fd);
printf(”received %d bytes, first word = %d\n”, recv_len,
(u_int)data_area[0]);