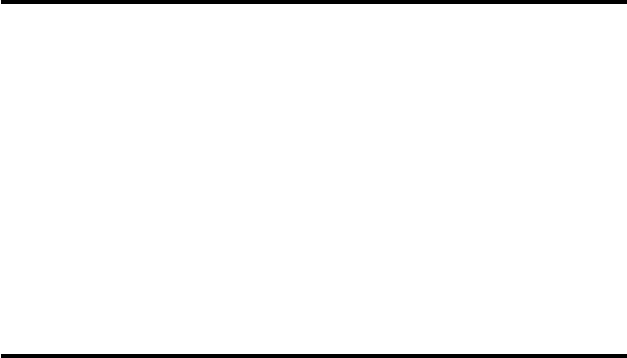
97
41 if (bind(sock, (struct sockaddr *)&name, sizeof(name)) < 0) {
42 perror(“binding datagram socket”);
43 exit(1);
44 }
45
46 /* Find assigned port value and print it out. Getsockname() gets
47 the local IP address and port number associated with a socket. */
48 length= sizeof(name);
49 if (getsockname(sock, (struct sockaddr *)&name, &length) < 0) {
50 perror(“getting socket name”);
51 exit(1);
52 }
53
54 printf(“Socket port #%d\n”, ntohs(name.sin_port));
55
56 while(1) {
57 /* Read from the socket */
58 if ((nbytes= read(sock, buf, 1024)) < 0) {
59 perror(“Receiving datagram packet”);
60 exit(2);
61 }
62 /* Print data to screen, not including Serial Adapter header */
63 buf[nbytes]= ‘\0’;
64 printf(“Data: —> %s\n”, buf+4);
65 }
66
67 }
Sample UDP Send Program
1 /********************************************************
2 * udpsend.c
3 * Sends a series of messages to a Serial Adapter using UDP.
4 *******************************************************/
5
6 #include <sys/types.h>
7 #include <sys/socket.h>
8 #include <netinet/in.h>
9 #include <netdb.h>
10 #include <stdio.h>
11
12 #define DATA “The sea is calm, the tide is full...”