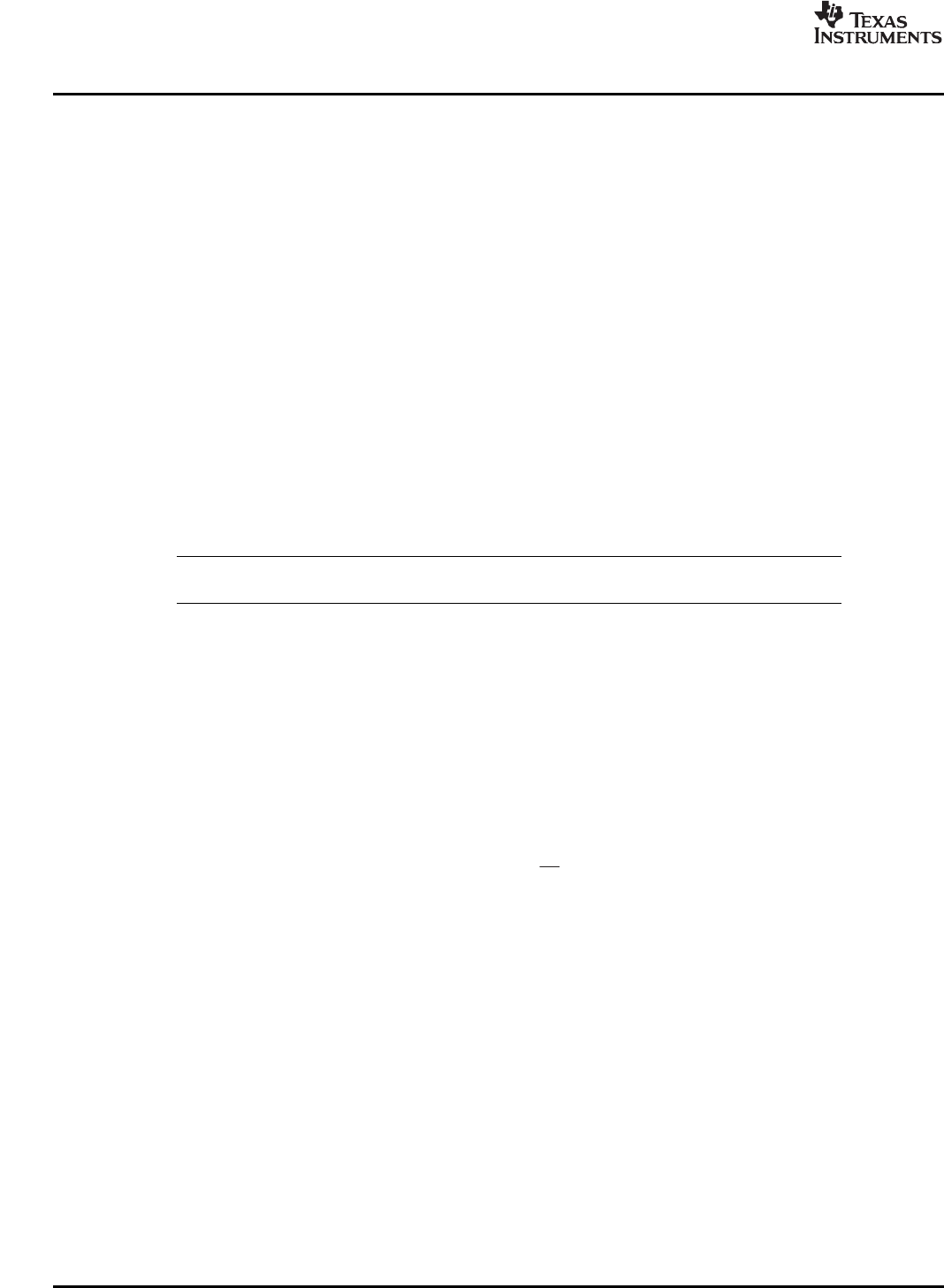
www.ti.com
2.2.4Example
y
n
+ x
n
* x
n*1
)
13
32
x
n*2
ThreadsandReentrancy
Thedefinitionofreentrantcodeoftenimpliesthatthecodedoesnotretain"state"information.Thatis,if
youinvokethecodewiththesamedataatdifferenttimes,bythesameorotherthread,itwillyieldthe
sameresults.Thisisnotalwaystrue,however.Howcanafunctionmaintainstateandstillbereentrant?
Considertherand()function.Perhapsabetterexampleisafunctionwithstatethatprotectsthatstateby
disablingschedulingarounditscriticalsections.Theseexamplesillustratesomeofthesubtletiesof
reentrantprogramming.
Thepropertyofbeingreentrantisafunctionofthethreadingmodel;afterall,beforeyoucandetermine
whethermultiplethreadscanuseaparticularfunction,youmustknowwhattypesofthreadsarepossible
inasystem.Forexample,ifthreadsarenotpreemptive,afunctionmayfreelyuseglobalvariablesifit
usesthemforscratchstorageonly;i.e.,itdoesnotassumethesevariableshaveanyvaluesuponentryto
thefunction.Inapreemptiveenvironment,however,useoftheseglobalvariablesmustbeprotectedbya
criticalsectionortheymustbepartofthecontextofeverythreadthatusesthem.
Althoughthereareexceptions,reentrancyusuallyrequiresthatalgorithms:
•onlymodifydataonthestackorinaninstance"object"
•treatglobalandstaticvariablesasread-onlydata
•neveremployselfmodifyingcode
Theserulescansometimesberelaxedbydisablingallinterrupts(andtherefore,disablingallthread
scheduling)aroundthecriticalsectionsthatviolatetherulesabove.Sincealgorithmsarenotpermittedto
directlymanipulatetheinterruptstateoftheprocessor,theallowedDSP/BIOSHWImodulefunctions(or
equivalentimplementations)mustbecalledtocreatethesecriticalsections.
Rule2
Allalgorithmsmustbereentrantwithinapreemptiveenvironment(includingtime-slicedpreemption).
Intheremainderofthissectionweconsiderseveralimplementationsofasimplealgorithm,digitalfiltering
ofaninputspeechdatastream,andshowhowthealgorithmcanbemadereentrantandmaintain
acceptablelevelsofperformance.Itisimportanttonotethat,althoughtheseexamplesarewritteninC,
theprinciplesandtechniquesapplyequallywelltoassemblylanguageimplementations.
Speechsignalsareoftenpassedthroughapre-emphasisfiltertoflattentheirspectrumpriortoadditional
processing.Pre-emphasisofasignalcanbeaccomplishedbyapplyingthefollowingdifferenceequation
totheinputdata:
Thefollowingimplementationisnotreentrantbecauseitreferencesandupdatestheglobalvariablesz0
andz1.Eveninanon-preemptiveenvironment,thisfunctionisnotreentrant;itisnotpossibletousethis
functiontooperateonmorethanonedatastreamsinceitretainsstateforaparticulardatastreamintwo
fixedvariables(z0andz1).
intz0=0,z1=0;/*previousinputvalues*/
voidPRE_filter(intinput[],intlength)
{
intI,tmp;
for(I=0;I<length;I++){
tmp=input[i]-z0+(13*z1+16)/32;
z1=z0;
z0=input[i];
input[i]=tmp;
}
}
Wecanmakethisfunctionreentrantbyrequiringthecallertosupplypreviousvaluesasargumentstothe
function.Thisway,PRE_filter1nolongerreferencesanyglobaldataandcanbeused,therefore,onany
numberofdistinctinputdatastreams.
18GeneralProgrammingGuidelinesSPRU352G–June2005–RevisedFebruary2007
SubmitDocumentationFeedback