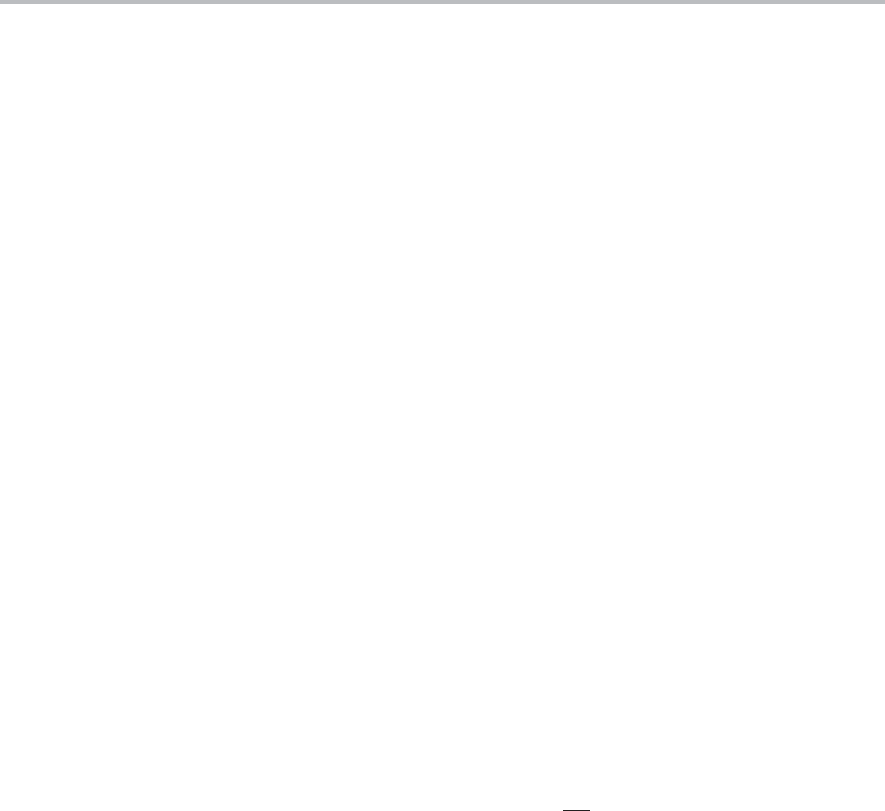
SPI Master Transfer in FIFO Mode using Interrupts
13-11
Serial Peripheral Interface (SPI)
13.8.2 SPI Master Transfer in FIFO Mode using Interrupts
Example 13−2. SPI Master Transfer in FIFO Mode using Interrupts
1 #include ”MSC1210.H”
2 void main(void)
3{
4 P1DDRH = 0x75; // P1.7,P1.5,P1.4=output P1.6=input
5 PDCON &= 0xFE; // Turn on SPI power
6 SPIRCON=0x83; // Flush RxBuf, RXlevel=4 or more
7 SPITCON=0xAA; // Flush TxBuf, DrvEnb=1, SCLK Enable=1 Txlevel=4 or less
8 SPISTRT=0x00; // Star address = 0
9 SPIEND= 0x08; // End address = 8
10 SPICON=0x36; // ClkDiv=001 (clk/4), dma=1, Order=0,M/S=1,CPHA=1,CPOL=0
11 AIE = 0x0C; // SPI Transmit IRQ and SPI Receive IRQ Enabled
12 AI=0; // Clear the external interrupt flag
13 EAI=1; // Enable the external interrupts.
14 while(1) { }
15 }
16 void monitor_isr() interrupt 6
17 {
18 if(AISTAT==0x04) {read_4_bytes();} // Checking for SPIRX IRQ
19 if(AISTAT==0x08) {send_4_bytes();} // Checking for SPITX IRQ
20 AI=0;
21 }
Example 13−2 is for a simple SPI master in FIFO mode using interrupts.
In line 4, the the port direction is set for the pins that are used by the SPI. Pins
P1.7 (SCLK pin), P1.5 (MOSI) and P1.4(SS
) are configured as outputs and pin
P1.6 (MISO) is configured as input because the device will be used in master
mode.
In line 5, the SPI module is powered up by writing to the PDCON.
In line 6 the Rxlevel is set to 4 and the RXBuffer content is flushed, if any exists.
Thus, SPIRXIRQ goes high whenever more than 4 bytes of data are received.
In line 7, the Txlevel is set to 4 and the TXBuffer content is also flushed, if any
exists. The data and clock lines are also enabled by setting bit 3 and bit 5, re-
spectively, of register SPITCON. The SPITXIRQ goes high if there are 4 or
fewer bytes to transmit.
In line 8, the SPISTRT SFR is cleared, i.e., the start address of the buffer is
at 0.
In line 9, the end address SPIEND is set to to 8, creating a buffer size of 8 bytes.
Line 10 sets the SPICON register. The clock is configured to run at clk/4 speed.
The other configuration settings are master mode, FIFO mode,
order = 0, CPHA = 1 and CPOL = 0.