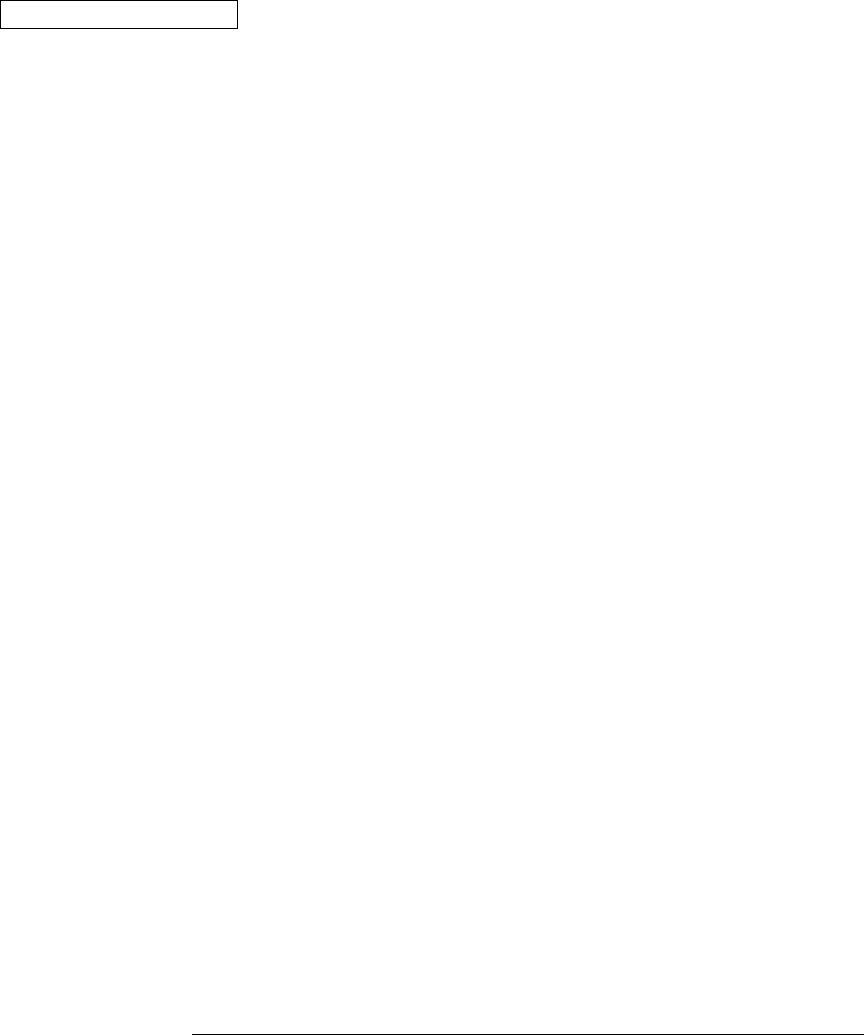
C / C++ Example: stat_reg.c
/* stat_reg.c
/*****************************************************************************
* Required: VISA library. *
* This program demonstrates the use of the 34970A Status Registers *
* for an alarm and Operation Complete (OPC) and for enabling and receiving *
* an SRQ interrupt. This program also shows how to configure a scan for *
* 10 readings on one channel. *
******************************************************************************/
#include <visa.h>
#include <stdio.h>
#include <string.h>
ViSession defaultRM; /* Resource manager id */
ViSession DataAcqu; /* Variable to identify an instrument */
char reply_string [256]= {0}; /* string returned from instrument */
double volt [10];
int index, count;
int srqFlag = {0};
/* Function prototypes for SRQ handler */
ViStatus _VI_FUNCH SRQ_handler(ViSession DataAcqu, ViEventType eventType,
ViEvent context,ViAddr userHdlr);
void main ()
{
/* Open communication with DataAcqu using GPIB address "9" */
viOpenDefaultRM (&defaultRM);
viOpen (defaultRM,"GPIB0::9::INSTR",VI_NULL,VI_NULL, &DataAcqu);
/* Reset instrument to power-on and clear the Status Byte */
viPrintf (DataAcqu, "*RST;*CLS\n");
/* Configure the Status Registers to generate an interrupt whenever an alarm
is detected on Alarm 1 or when the operation is complete */
viPrintf (DataAcqu, "STATUS:ALARM:ENABLE 1\n"); /* Enable Alarm 1 */
viPrintf (DataAcqu, "*ESE 1\n"); /* Enable the Operation Complete bit */
/* Enable Status Byte Register bit 1 (2) and 5 (32) for SRQ */
viPrintf (DataAcqu, "*SRE 34\n");
/* Enable the interrupt handler for SRQ from the instrument */
viInstallHandler(DataAcqu, VI_EVENT_SERVICE_REQ, SRQ_handler, (ViAddr)10);
viEnableEvent(DataAcqu,VI_EVENT_SERVICE_REQ, VI_HNDLR, VI_NULL);
/* Configure the instrument to take 10 dc voltage readings on channel 103.
Set the alarm and set SRQ if the voltage is greater than 5 volts.*/
viPrintf (DataAcqu, "CONF:VOLT:DC 10,(@103)\n");
viPrintf (DataAcqu, "TRIG:SOURCE TIMER\n");
viPrintf (DataAcqu, "TRIG:TIMER 1\n");
viPrintf (DataAcqu, "TRIG:COUNT 10\n");
viPrintf (DataAcqu, "CALC:LIMIT:UPPER 5,(@103)\n");
viPrintf (DataAcqu, "CALC:LIMIT:UPPER:STATE ON,(@103)\n");
viPrintf (DataAcqu, "OUTPUT:ALARM1:SOURCE (@103)\n");
viPrintf (DataAcqu, "INIT;*OPC\n");
/* Wait for the instrument to complete its operations so waste time
and stay in the program in case there is an SRQ */
Continued on next page
Chapter 7 Application Programs
Example Programs for C and C++
330